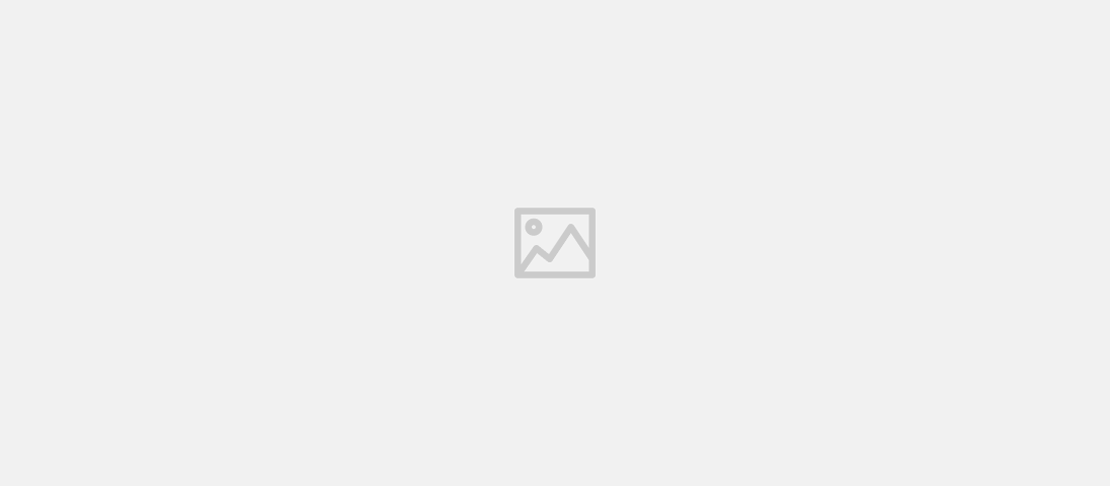
Enhancements to dbg in Elixir 1.18
- Daniel Kukula
- Development , Tutorial
- December 22, 2024
Table of Contents
Elixir 1.18 added some interesting features, but one that went under the radar was extended support for dbg
. In 1.17 when you had this code:
a = 1
b = 2
if a + b == 3 do
:equal
else
:non_equal
end
|> dbg
It evaluated to:
if a + b == 3 do
:equal
else
:non_equal
end #=> :equal
In 1.18 this was aligned with what case
and cond
does:
Case argument:
1 + 2 #=> 3
Case expression (clause #1 matched):
case 1 + 2 do
3 -> :equal
_ -> :non_equal
end #=> :equal
if
result in 1.18:
If condition:
a + b == 3 #=> true
If expression:
if a + b == 3 do
:equal
else
:non_equal
end #=> :equal
If this is not enough you can wrap your code in brackets and pass it to dbg:
(
input = %{a: 1, b: 2}
c = Map.get(input, :c, 20)
if input[:a] + c == 3 do
:equal
else
:non_equal
end
)
|> dbg
Results in displaying results for every expression:
Code block:
(
input = %{a: 1, b: 2} #=> %{a: 1, b: 2}
c = Map.get(input, :c, 20) #=> 20
if input[:a] + c == 3 do
:equal
else
:non_equal
end #=> :non_equal
)
Last missing piece is the with
macro which is sometimes a nightmare to debug, in Elixir 1.18 we have support for that:
with input = %{a: 1, b: 2},
{:ok, c} <- Map.fetch(input, :c),
3 <- input[:a] + c do
:equal
else
_ -> :non_equal
end
|> dbg
The result here is:
With clauses:
%{a: 1, b: 2} #=> %{a: 1, b: 2}
Map.fetch(input, :c) #=> :error
With expression:
with input = %{a: 1, b: 2},
{:ok, c} <- Map.fetch(input, :c),
3 <- input[:a] + c do
:equal
else
_ -> :non_equal
end #=> :non_equal
This should make your debugging journey easier. If you are stuck in older Elixir versions you can install a package that backports this functionality dbg_mate. Thanks for your attention.